1293. Shortest Path in a Grid with Obstacles Elimination
1293. Shortest Path in a Grid with Obstacles Elimination
Description
You are given an m x n
integer matrix grid
where each cell is either 0
(empty) or 1
(obstacle). You can move up, down, left, or right from and to an empty cell in one step .
Return the minimum number of steps to walk from the upper left corner (0, 0)
to the lower right corner (m - 1, n - 1)
given that you can eliminate at most k
obstacles. If it is not possible to find such walk return -1
.
Example 1:
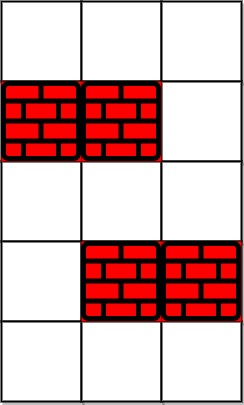
1 | Input: grid = [[0,0,0],[1,1,0],[0,0,0],[0,1,1],[0,0,0]], k = 1 |
Example 2:
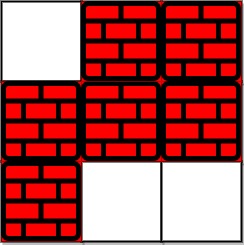
1 | Input: grid = [[0,1,1],[1,1,1],[1,0,0]], k = 1 |
Constraints:
m == grid.length
n == grid[i].length
1 <= m, n <= 40
1 <= k <= m * n
grid[i][j]
is either0
or1
.grid[0][0] == grid[m - 1][n - 1] == 0
Hints/Notes
- 2025/02/18 Q3
- bfs
- Leetcode solution
Solution
Language: C++
1 | class Solution { |
A* algorithm:
1 | class Solution { |