2268. Minimum Number of Keypresses
2268. Minimum Number of Keypresses
Description
You have a keypad with 9
buttons, numbered from 1
to 9
, each mapped to lowercase English letters. You can choose which characters each button is matched to as long as:
- All 26 lowercase English letters are mapped to.
- Each character is mapped to by exactly
1
button. - Each button maps to at most
3
characters.
To type the first character matched to a button, you press the button once. To type the second character, you press the button twice, and so on.
Given a string s
, return the minimum number of keypresses needed to type s
using your keypad.
Note that the characters mapped to by each button, and the order they are mapped in cannot be changed.
Example 1:
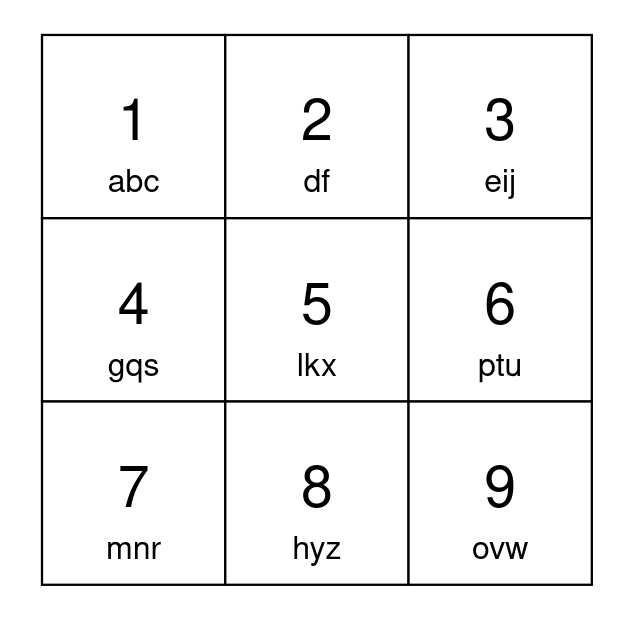
1 | Input: s = "apple" |
Example 2:
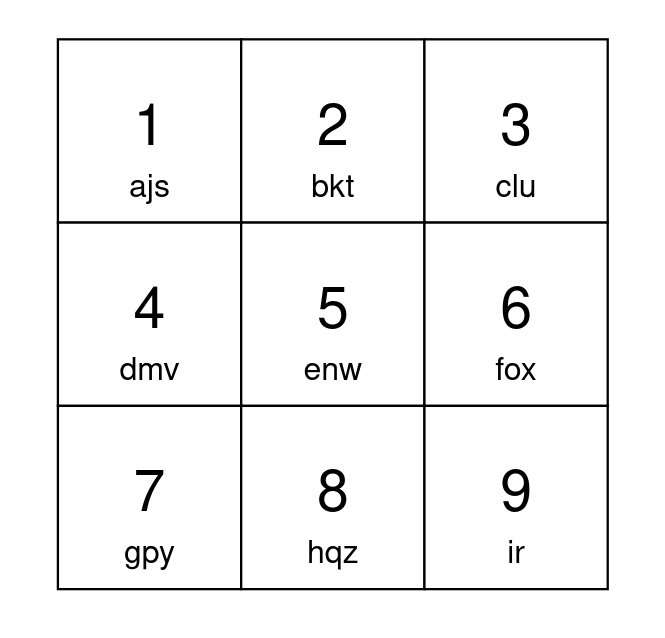
1 | Input: s = "abcdefghijkl" |
Constraints:
1 <= s.length <= 10^5
s
consists of lowercase English letters.
Hints/Notes
- 2025/02/08 Q2
- sorting
- No solution from 0x3F or Leetcode
Solution
Language: C++
1 | class Solution { |