1232. Check If It Is a Straight Line
1232. Check If It Is a Straight Line
Description
You are given an arraycoordinates
, coordinates[i] = [x, y]
, where [x, y]
represents the coordinate of a point. Check if these pointsmake a straight line in the XY plane.
Example 1:
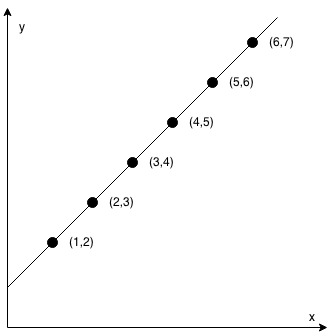
1 | Input: coordinates = [[1,2],[2,3],[3,4],[4,5],[5,6],[6,7]] |
Example 2:
1 | Input: coordinates = [[1,1],[2,2],[3,4],[4,5],[5,6],[7,7]] |
Constraints:
2 <=coordinates.length <= 1000
coordinates[i].length == 2
-10^4 <=coordinates[i][0],coordinates[i][1] <= 10^4
coordinates
contains no duplicate point.
Hints/Notes
- 2025/03/12 Q3
- math
- 0x3Fâs solution
Solution
Language: C++
1 | class Solution { |