545. Boundary of Binary Tree
Description
The boundary of a binary tree is the concatenation of the root , the left boundary , the leaves ordered from left-to-right, and the reverse order of the right boundary .
The left boundary is the set of nodes defined by the following:
- The root node’s left child is in the left boundary. If the root does not have a left child, then the left boundary is empty .
- If a node in the left boundary and has a left child, then the left child is in the left boundary.
- If a node is in the left boundary, has no left child, but has a right child, then the right child is in the left boundary.
- The leftmost leaf is not in the left boundary.
The right boundary is similar to the left boundary , except it is the right side of the root’s right subtree. Again, the leaf is not part of the right boundary , and the right boundary is empty if the root does not have a right child.
The leaves are nodes that do not have any children. For this problem, the root is not a leaf.
Given the root
of a binary tree, return the values of its boundary .
Example 1:
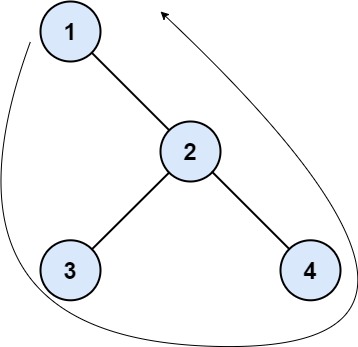
1 | Input: root = [1,null,2,3,4] |
Example 2:
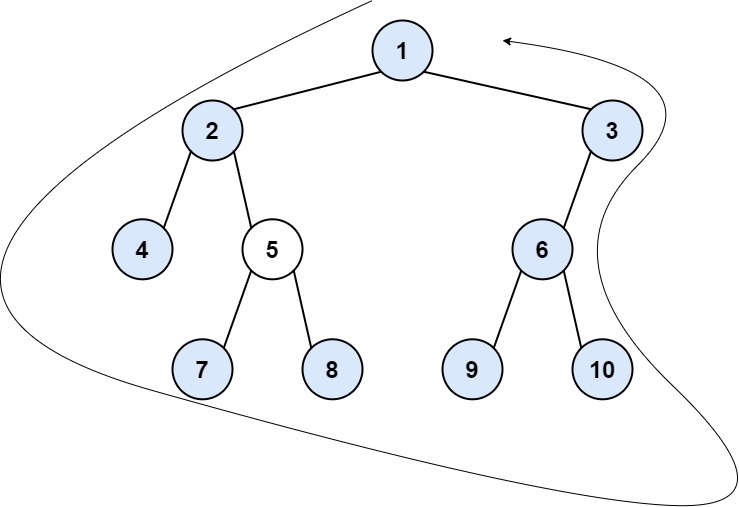
1 | Input: root = [1,2,3,4,5,6,null,null,null,7,8,9,10] |
Constraints:
- The number of nodes in the tree is in the range
[1, 10^4]
. -1000 <= Node.val <= 1000
Hints/Notes
- 2025/02/17 Q1
- binary tree
- Leetcode solution
Solution
Language: C++
1 | /** |