1424. Diagonal Traverse II
Description
Given a 2D integer array nums
, return all elements of nums
in diagonal order as shown in the below images.
Example 1:
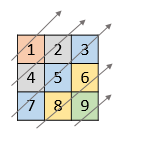
1 | Input: nums = [[1,2,3],[4,5,6],[7,8,9]] |
Example 2:
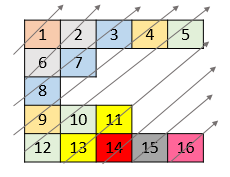
1 | Input: nums = [[1,2,3,4,5],[6,7],[8],[9,10,11],[12,13,14,15,16]] |
Constraints:
1 <= nums.length <= 10^5
1 <= nums[i].length <= 10^5
1 <= sum(nums[i].length) <= 10^5
1 <= nums[i][j] <= 10^5
Hints/Notes
- 2025/01/30
- bfs
- Leetcode solution(checked)
Solution
Language: C++
1 | class Solution { |