2271. Maximum White Tiles Covered by a Carpet
2271. Maximum White Tiles Covered by a Carpet
Description
You are given a 2D integer array tiles
where tiles[i] = [li, ri] represents that every tile j
in the range li <= j <= ri is colored white.
You are also given an integer carpetLen
, the length of a single carpet that can be placed anywhere .
Return the maximum number of white tiles that can be covered by the carpet.
Example 1:
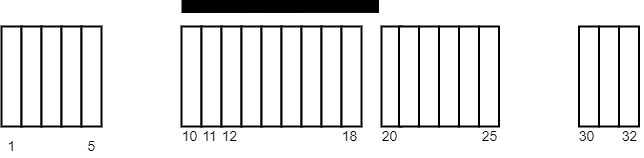
1 | Input: tiles = [[1,5],[10,11],[12,18],[20,25],[30,32]], carpetLen = 10 |
Example 2:
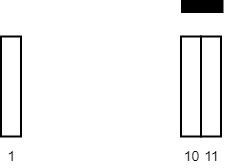
1 | Input: tiles = [[10,11],[1,1]], carpetLen = 2 |
Constraints:
1 <= tiles.length <= 5 * 10^4
tiles[i].length == 2
s- 1 <= li <= ri <= 10^9
1 <= carpetLen <= 10^9
- The
tiles
are non-overlapping .
Hints/Notes
- 2025/01/13
- sort and sliding window
- 0x3Fâs solution(checked)
Solution
Language: C++
1 | class Solution { |