286. Walls and Gates
Description
You are given an m x n
grid rooms
initialized with these three possible values.
-1
A wall or an obstacle.0
A gate.INF
Infinity means an empty room. We use the value2^31 - 1 = 2147483647
to representINF
as you may assume that the distance to a gate is less than2147483647
.
Fill each empty room with the distance to its nearest gate. If it is impossible to reach a gate, it should be filled with INF
.
Example 1:
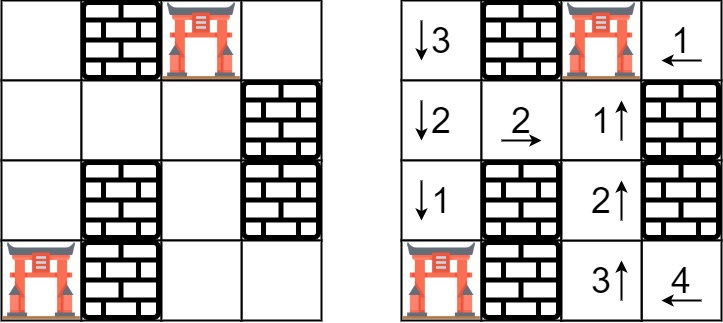
1 | Input: rooms = [[2147483647,-1,0,2147483647],[2147483647,2147483647,2147483647,-1],[2147483647,-1,2147483647,-1],[0,-1,2147483647,2147483647]] |
Example 2:
1 | Input: rooms = [[-1]] |
Constraints:
m == rooms.length
n == rooms[i].length
1 <= m, n <= 250
rooms[i][j]
is-1
,0
, or2^31 - 1
.
Hints/Notes
- 2025/01/05
- bfs
- premium
Solution
Language: C++
1 | class Solution { |