17. Letter Combinations of a Phone Number
17. Letter Combinations of a Phone Number
Description
Given a string containing digits from 2-9
inclusive, return all possible letter combinations that the number could represent. Return the answer in any order .
A mapping of digits to letters (just like on the telephone buttons) is given below. Note that 1 does not map to any letters.
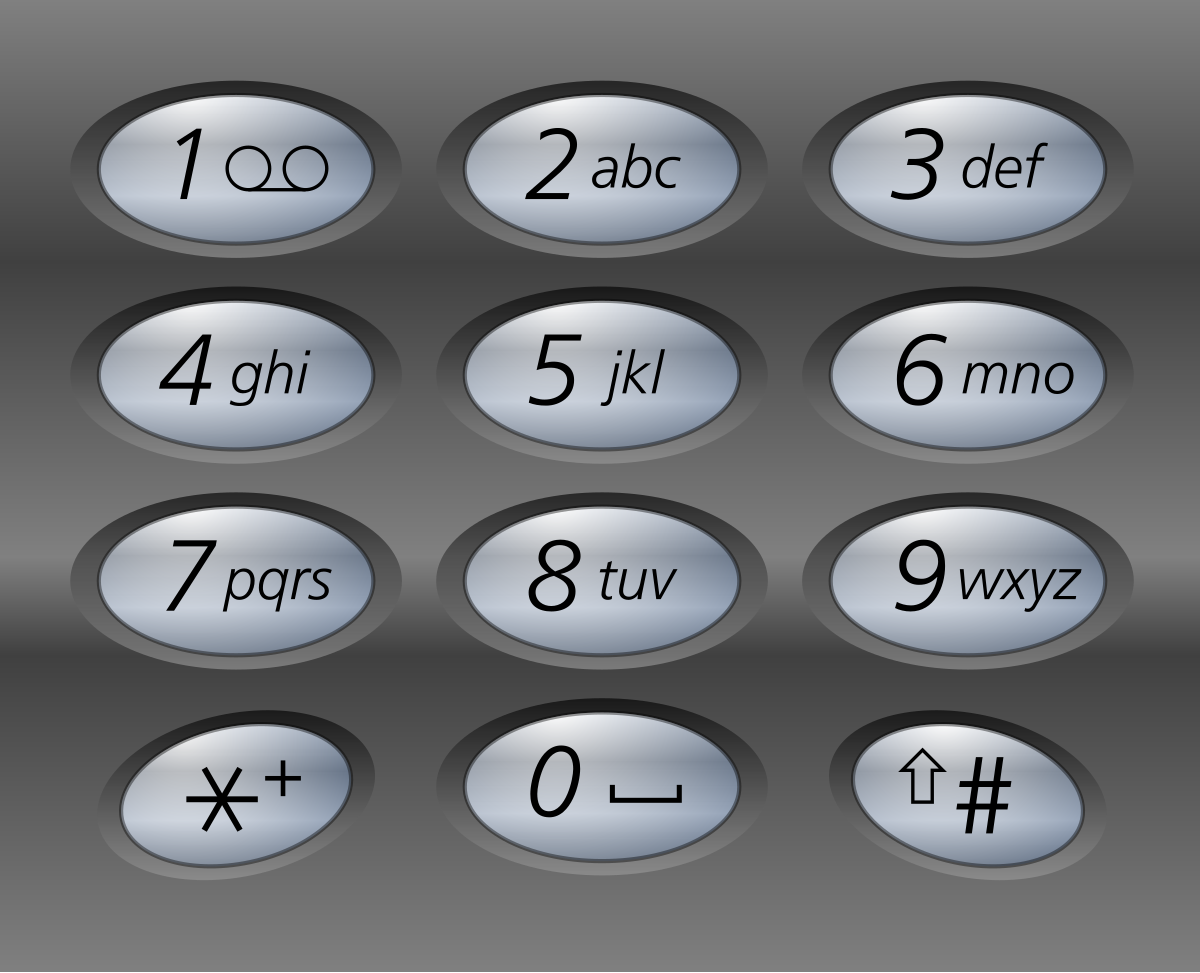
Example 1:
1 | Input: digits = "23" |
Example 2:
1 | Input: digits = "" |
Example 3:
1 | Input: digits = "2" |
Constraints:
0 <= digits.length <= 4
digits[i]
is a digit in the range['2', '9']
.
Hints/Notes
- 2024/12/18
- backtracking or for loop
- 0x3Fâs solution(checked)
Solution
Language: C++
Backtracking
1 | class Solution { |
1 | class Solution { |