3212. Count Submatrices With Equal Frequency of X and Y
3212. Count Submatrices With Equal Frequency of X and Y
Description
Given a 2D character matrix grid
, where grid[i][j]
is either 'X'
, 'Y'
, or '.'
, return the number of submatrices that contain:
grid[0][0]
- an equal frequency of
'X'
and'Y'
. - at least one
'X'
.
Example 1:
1 | Input: grid = [["X","Y","."],["Y",".","."]] |
Explanation:
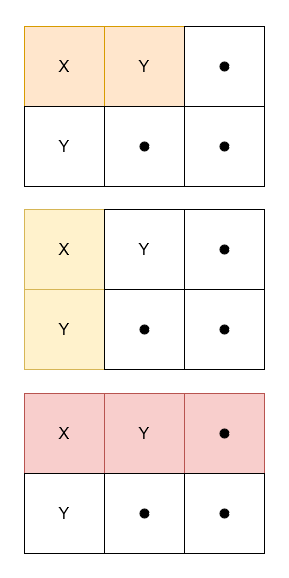
Example 2:
1 | Input: grid = [["X","X"],["X","Y"]] |
Explanation:
No submatrix has an equal frequency of 'X'
and 'Y'
.
Example 3:
1 | Input: grid = [[".","."],[".","."]] |
Explanation:
No submatrix has at least one 'X'
.
Constraints:
1 <= grid.length, grid[i].length <= 1000
grid[i][j]
is either'X'
,'Y'
, or'.'
.
Hints/Notes
- preSum
- Weekly Contest 405
Solution
Language: C++
1 | class Solution { |