1602. Find Nearest Right Node in Binary Tree
1602. Find Nearest Right Node in Binary Tree
Description
Given the root
of a binary tree and a node u
in the tree, return the nearest node on the same level that is to the right of u
, or return null
if u
is the rightmost node in its level.
Example 1:
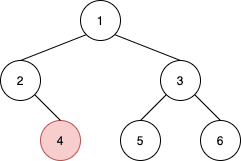
1 | Input: root = [1,2,3,null,4,5,6], u = 4 |
Example 2:
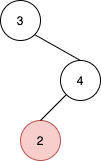
1 | Input: root = [3,null,4,2], u = 2 |
Constraints:
- The number of nodes in the tree is in the range
[1, 10^5]
. 1 <= Node.val <= 10^5
- All values in the tree are distinct .
u
is a node in the binary tree rooted atroot
.
Hints/Notes
- use targetDepth as a proxy to show the node has been found
Solution
Language: C++
1 | /** |